Visual C++ Example:
MFC Bitmap Button Source
Code (CButton)
|
Introduction
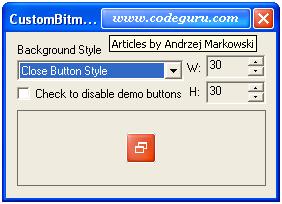
CCustomBitmapButton
is MFC control derived
from the CWnd class. The button has two parts: a
background and a foreground. If the operating system is
WinXP and XP Themes are enabled the background is a bitmap
loaded from the current active theme resource file (I use
similar technique to draw the scroll buttons in the
CCustomTabCtrl control); otherwise, the "DrawFrameControl"
function is used to draw the button background. The
foreground is a user-defined monochrome bitmap (glyph)
drawn transparently on the button background.
Supported
features:
-
Standard
or XP Themes view
-
12
predefined background styles
-
User-defined
foregrounds (bitmap glyphs)
-
Button
states supported: "NORMAL","HOT","PRESSED",
and "DISABLED"
-
Buttons
can be created in the caption bar area
-
Dialog,
SDI and MDI support for the caption buttons
-
Ficker-free
drawing
-
Built-in
tooltips
Using the
Code
To integrate
the CCustomBitmapButton
class into your application as a caption frame, please
follow the steps below:
-
Add
ThemeUtil.h, ThemeUtil.cpp, CustomBitmapButton.h,
CustomBitmapButton.cpp, Tmschema.h, and Schemadef.h to
your project.
-
Include
CustomBitmapButton.h to the appropriate header
file—usually the dialog class header where the
CCustomBitmapButton class is used.
#include "CustomBitmapButton.h"
-
Declare
m_ctrlCaptionFrame object of type CCustomBitmapButton
in your dialog header.
class CCustomBitmapButtonDemoDlg : CDialog
{
......
private:
CCustomBitmapButton m_ctrlCaptionFrame;
};
-
Create
the caption frame.
In your
dialog's OnInitDialog, add the following code:
m_ctrlCaptionFrame.CreateCaptionFrame(this,IDR_MAINFRAME);
-
After
creating the caption frame, add as many buttons
as you need.
To add
caption buttons,
call AddCaptionButton in your dialog's OnInitDialog:
m_ctrlCaptionFrame.AddCaptionButton(CRect(0,0,0,0),1,
CBNBKGNDSTYLE_CLOSE, FALSE);
m_ctrlCaptionFrame.AddCaptionButton(CRect(0,0,150,0),2,
CBNBKGNDSTYLE_CAPTION, TRUE);
CCustomBitmapButton* pBn1 =
m_ctrlCaptionFrame.GetCaptionButtonPtr(1);
if(pBn1)
{
CBitmap bmpGlyph1;
bmpGlyph1.LoadBitmap(IDB_GLYPH1);
pBn1->SetGlyphBitmap(bmpGlyph1);
pBn1->SetTooltipText(_T("Double click to close the
window, Right click to
display the popup menu"));
}
CCustomBitmapButton* pBn2 =
m_ctrlCaptionFrame.GetCaptionButtonPtr(2);
if(pBn2)
{
CBitmap bmpGlyph2;
bmpGlyph2.LoadBitmap(IDB_GLYPH2);
pBn2->SetGlyphBitmap(bmpGlyph2);
pBn2->SetTooltipText(_T("Articles by Andrzej
Markowski"));
}
-
Process
WM_NOTIFY messages from the caption buttons in your
dialog class. As users click a button,
the button sends notification messages (NM_CLICK,
NM_RCLICK, NM_DBLCLK, and NM_RDBLCLK) to its parent
window. Handle these messages if you want to do
something in response.
BEGIN_MESSAGE_MAP(CCustomBitmapButtonDemoDlg, CDialog)
ON_NOTIFY(NM_DBLCLK, 1, OnBnDblClickedCaptionbn1)
ON_NOTIFY(NM_RCLICK, 1, OnBnRClickedCaptionbn1)
ON_NOTIFY(NM_CLICK, 2, OnBnClickedCaptionbn2)
END_MESSAGE_MAP()
....
void CCustomBitmapButtonDemoDlg::
OnBnDblClickedCaptionbn1(NMHDR * pNotifyStruct,
LRESULT * result)
{
CPoint pt;
::GetCursorPos(&pt);
PostMessage(WM_SYSCOMMAND,SC_CLOSE,MAKEWORD(pt.x,pt.y));
}
....
-
Don't
forget to destroy the caption frame; otherwise, you
will have memory leaks.
void CCustomBitmapButtonDemoDlg::OnDestroy()
{
m_ctrlCaptionFrame.DestroyCaptionFrame();
CDialog::OnDestroy();
}
CCustomBitmapButton
Class Members
Construction/Destruction
Attributes
Operations
Notification Messages
Error Codes
Construction/Destruction
Attributes
Operations
CCustomBitmapButton::CCustomBitmapButton
CCustomBitmapButton(
);
Remarks
Call this
function to construct a CCustomBitmapButton object.
CCustomBitmapButton::Create
BOOL Create(
DWORD dwStyle, const RECT&
rect, CWnd* pParentWnd,
UINT nID );
Return
Value
TRUE
if initialization of the object was successful; otherwise,
FALSE.
Parameters
dwStyle—Specifies
the button style.
rect—Specifies
the button size and position. It can be either a CRect
object or a RECT structure.
pParentWnd—Specifies
the button parent window.
nID—Specifies
the button ID.
Remarks
Creates a
bitmap button and attaches it to an instance of a CCustomBitmapButton
object.
CCustomBitmapButton::CreateCaptionFrame
int CreateCaptionFrame(
CWnd* pCaptionWnd, int nIDIcon
);
Return
Value
CBNERRR_NOERROR
if successful; otherwise, CBNERRR_ERRORCODE.
Parameters
pCaptionWnd—Specifies
the caption bar owner window.
nIDIcon—Specifies
the caption icon resource id.
Remarks
Creates a
caption frame.
CCustomBitmapButton::DestroyCaptionFrame
int DestroyCaptionFrame();
Return
Value
CBNERRR_NOERROR
if successful; otherwise, CBNERRR_ERRORCODE.
Remarks
Destroys a
caption frame.
CCustomBitmapButton::GetCaptionButtonPtr
CCustomBitmapButton*
GetCaptionButtonPtr( int nButtonID )
const;
Return
Value
Pointer to
the CCustomBitmapButton object if successful;
otherwise, NULL.
Parameters
nButtonID—The
ID of the caption button.
Remarks
Call this
function to retrieve the pointer to the caption button.
CCustomBitmapButton::SetTooltipText
int SetTooltipText(
CString sText );
Return
Value
CBNERRR_NOERROR
if successful; otherwise, CBNERRR_ERRORCODE.
Parameters
sText—Pointer
to a string object that contains the new tooltip text.
Remarks
This
function changes the tooltip text of a button.
CCustomBitmapButton::GetBkStyle
int GetBkStyle();
Return
Value
Returns the
button background style for this CCustomBitmapButton
object.
Remarks
Call this
function to retrieve information about the button control
background style.
CCustomBitmapButton::SetBkStyle
int SetBkStyle(
int nBkStyle );
Return
Value
CBNERRR_NOERROR
if successful; otherwise, CBNERRR_ERRORCODE.
Parameters
nBkStyle—Specifies
the button background style.
Supported
background styles:
-
CBNBKGNDSTYLE_CAPTION—frame
buttons
-
CBNBKGNDSTYLE_CLOSE
-
CBNBKGNDSTYLE_MDI
-
CBNBKGNDSTYLE_COMBO—combobox
dropdown button
-
CBNBKGNDSTYLE_SPINUP—spin
buttons
-
CBNBKGNDSTYLE_SPINDN
-
CBNBKGNDSTYLE_SPINUPHOR
-
CBNBKGNDSTYLE_SPINDNHOR
-
CBNBKGNDSTYLE_SCROLLUP—scrollbar
buttons
-
CBNBKGNDSTYLE_SCROLLDOWN
-
CBNBKGNDSTYLE_SCROLLLEFT
-
CBNBKGNDSTYLE_SCROLLRIGHT
Remarks
Changes the
background style of a button.
CCustomBitmapButton::GetGlyphBitmap
HBITMAP
GetGlyphBitmap();
Return
Value
A handle to
a bitmap. NULL if no bitmap is previously
specified.
Remarks
Call this
member function to get the handle of a glyph bitmap that
is associated with a button.
CCustomBitmapButton::SetGlyphBitmap
int SetGlyphBitmap(
HBITMAP hBmpGlyph );
Return
Value
CBNERRR_NOERROR
if successful; otherwise, CBNERRR_ERRORCODE.
Parameters
hBmpGlyph—The
handle of a bitmap.
Remarks
Call this
member function to associate a new glyph bitmap with the
button.
CCustomBitmapButton::EnableWindow
int EnableWindow(
BOOL bEnable = TRUE);
Return
Value
CBNERRR_NOERROR
if successful; otherwise, CBNERRR_ERRORCODE.
Parameters
bEnable—Specifies
whether the given window is to be enabled or disabled. If
this parameter is TRUE, the window will be enabled.
If this parameter is FALSE, the window will be
disabled.
Remarks
Call this
function to enable or disable a button control.
CCustomBitmapButton::AddCaptionButton
int
AddCaptionButton( LPCRECT lpRect, UINT nID,
int nBkStyle, BOOL fUserDefWidth
);
Return
Value
CBNERRR_NOERROR
if successful; otherwise, CBNERRR_ERRORCODE.
Parameters
lpRect—Specifies
the button width if fUserDefWidth is true;
otherwise, ignored.
nID—Specifies
the button ID.
nBkStyle—Specifies
the button background style.
fUserDefWidth—If
TRUE—user defined button width.
Remarks
Call this
function to insert a new button in an existing
caption bar.
Notification
Messages
The
following notification codes are supported by the button
control:
-
NM_CLICK—User
clicked left mouse button in the control.
-
NM_RCLICK—User
clicked right mouse button in the control.
-
NM_DBLCLK—User
double clicked left mouse button in the
control.
-
NM_RDBLCLK—User
double clicked right mouse button in the
control.
Error
Codes
The
following errors can be returned by the button
control functions:
-
CBNERRR_NOERROR—Operation
succesful.
-
CBNERR_OUTOFMEMORY—Function
call failed because there was not enough memory
available.
-
CBNERR_INCORRECTPARAMETER—Function
call failed because an incorrect function parameter
was specified.
-
CBNERR_INCORRECTBUTTONSTYLE—Function
call failed because an incorrect button style was
specified.
-
CBNERR_INCORRECTFRAMESTYLE—Function
call failed because the WS_CAPTION style was not
specified.
-
CBNERR_INCORRECTFUNCCALL—Incorrect
function call (for example: ob.SetTooltipText(...)
where ob is an instance of the caption frame object).
-
CBNERR_CREATEBUTTONFAILED—Function
call failed because creation of the button control
failed.
-
CBNERR_CREATETOOLTIPCTRLFAILED—Function
call failed because creation of the tooltip control
failed.
-
CBNERR_COPYIMAGEFAILED—Function
call failed because copying of the glyph image failed.
-
CBNERR_SETWINDOWLONGFAILED—Call
to the function "SetWindowLong" failed.
-
CBNERR_FRAMEALREADYCREATED—Function
call failed because the caption frame was already
created.
-
CBNERR_FRAMENOTCREATED—Function
call failed because the caption frame was not created.
Downloads
CustomBitmapButtonDemo.zip
- Download source VC++
demo project - 40 Kb
CustomBitmapButtonDemo_exe.zip
- Download sample
demo executable - 26 Kb
|